Animation in Three.js with Delta Time
release date :
- Coding
Hello from the Engineer Team!
Today, let’s delve into the world of Three.js.
To be more specific, let’s discuss the art of animating in Three.js by exploring the concepts of elapsedTime()
and delta time.
Table of Contents
Tick Function
If you’re not familiar with Three.js, the tick function is where all the updates happen.
Whether you’re resizing your window or animating objects in your scene, the tick function ensures that your canvas gets updated with a fresh render.
const tick = () =>
{
renderer.render(scene,camera)
window.requestAnimationFrame(tick)
}
tick()
elapsedTime()
But how do you determine the frequency or extent of updates handled by the tick function?
Here’s our introduction to a useful function called elapsedTime()
.
This function begins counting up once your canvas is loaded.
You can then incorporate this elapsed time into any elements you wish to animate.
const clock = new THREE.Clock()
const tick = () =>
{
const elapsedTime = clock.getElapsedTime()
renderer.render(scene,camera)
window.requestAnimationFrame(tick)
}
tick()
Goodbye, cube.
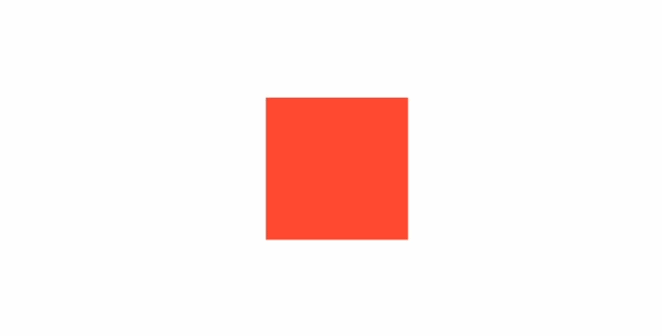
You have the flexibility to enhance your animation by incorporating functions such as sine or cosine.
These functions help ensure that the object remains within a specific range, adding a dynamic and controlled movement to your visual elements.
See the Pen hybrid scrolling by bibomato (@bibomato) on CodePen.
The Problem with elapsedTime()
The primary challenge with elapsedTime()
lies in its continuously increasing nature.
While this suffices for many applications, introducing GSAP and ScrollTrigger into the equation reveals a potential issue.
For example, let’s increase the speed of rotation based on the scroll position.
See the Pen hybrid scrolling by bibomato (@bibomato) on CodePen.
When scrolling up and down the page, you may observe the cube moving more rapidly or experiencing skips.
This erratic behavior is a result of tying the elapsedTime()
value to the scroll position.
When scrolling up, elapsedTime()
continues to increase but also moves backward simultaneously.
While this concept is intriguing, visually, it can be disconcerting.
See the Pen hybrid scrolling by bibomato (@bibomato) on CodePen.
Delta Time
This is where delta time comes to the rescue.
Delta time represents the time unit between each update of elapsedTime()
.
Delta time is computed by subtracting the current time from the previous time when the tick function was last updated.
const clock = new THREE.Clock()
let previousTime = 0
const tick = () =>
{
const elapsedTime = clock.getElapsedTime()
// Delta Time - デルタタイム
const deltaTime = elapsedTime - previousTime
previousTime = elapsedTime
// Animation - アニメーション
mesh.rotation.y += deltaTime * speed.value
renderer.render(scene, camera)
window.requestAnimationFrame(tick)
}
tick()
In the realm of animation, you can conceptualize delta time as a frame.
It provides a more controlled and consistent measure, addressing the issue of erratic behavior tied to scrolling movements.
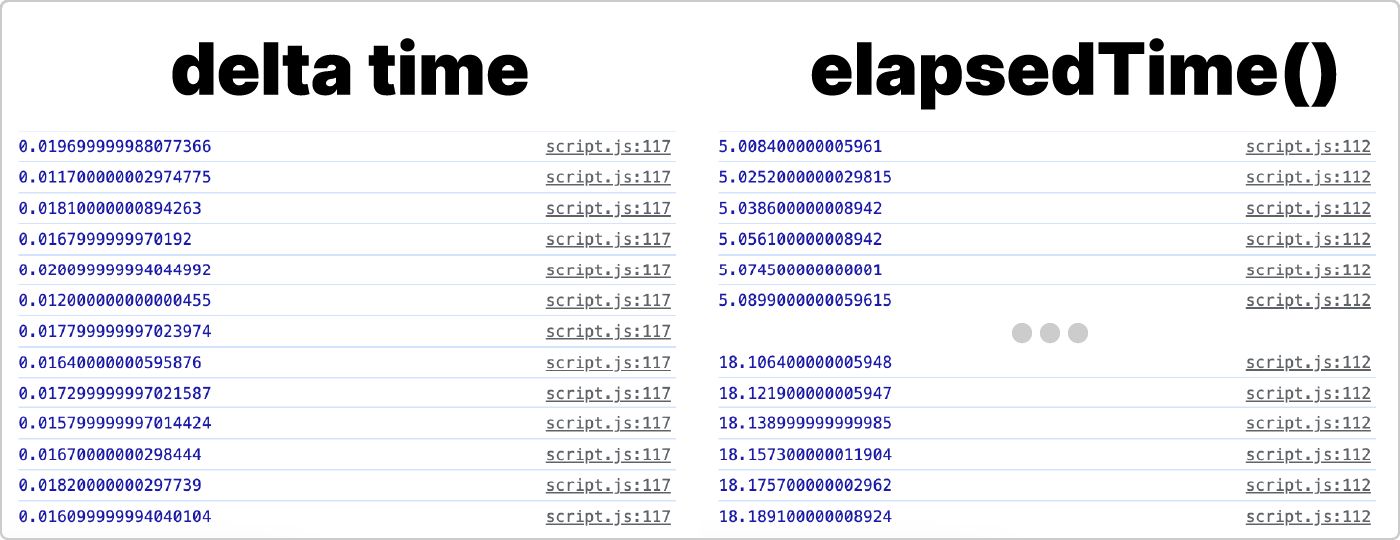
By integrating delta time into the scroll animation, the animation’s value will now smoothly scale in correlation with the scroll position.
This addition ensures a gradual and proportional adjustment, enhancing the overall visual experience as you navigate through the scroll.
See the Pen hybrid scrolling by bibomato (@bibomato) on CodePen.
getDelta()
Three.js conveniently provides a getDelta()
function, streamlining the process of obtaining the delta time.
This built-in function simplifies the calculation, making it more accessible and efficient for managing time-based updates in your animations.
const clock = new THREE.Clock()
const tick = () =>
{
// Delta Time - デルタタイム
const deltaTime = clock.getDelta()
mesh.rotation.y += deltaTime * speed.value
renderer.render(scene,camera)
window.requestAnimationFrame(tick)
}
tick()
Conclusion
Delta time stands as a crucial concept in the realm of graphics programming.
Its incorporation is instrumental in achieving smoother and more lifelike animations in your projects.
Moreover, it has the potential to save individuals valuable hours of frustration in navigating the intricacies of animation timing.
Until next time,
-M